At the end of this tutorial we will have a grid like the following
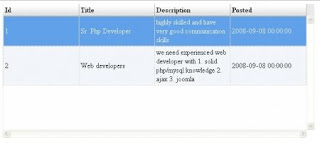
So let’s feel the power of dojo in zend.
First execute the following query for creating a table.
CREATE TABLE 'jobs' (
'id' int(11) NOT NULL auto_increment,
'title' varchar(50) NOT NULL,
'description' text,
'posted_date' datetime default NULL,
PRIMARY KEY ('id')
)
This will create a table in your database.
Create a model in your models directory and write the following code in it.
class Jobs extends Zend_Db_Table_Abstract
{
protected $_name='jobs';
public function getJobsData()
{
$select = $this->_db->select()
->from($this->_name);
$results = $this->getAdapter()->fetchAll($select);
return $results;
}
}
and save this table as Jobs.php
In the code above, we first extend our model from Zend_Db_Table_Abstract, define its name and define our custom function getJobsData() that fetch all the rows in the jobs table. We return the results as an array.
Next create a controller JobsController in you controller’s directory and place the following code in it.
<?php
class JobsController extends Zend_Controller_Action
{
public function viewAction()
{
}
public function recordsAction()
{
$jobs = new Jobs();
$data= $jobs->getJobsData();
$dojoData= new Zend_Dojo_Data(’id’,$data,’id’);
echo $dojoData->toJson();
exit;
}
}
In the above code we create two action view and records. We have placed nothing in the view action. In the recordsAction, we create an instance of Jobs model class.and then call its getJobsData() method which will give us an array of records. Next we create an instance of Zend_Dojo_Data() and give the fetched data to it. And finally we echo the data converted it into the Json.
We don’t need to create a view for the records action because we have placed exit it the end of this action which will stop rendering of the view. As we will call this action form our grid view template page so no need to define phtml file for it.
However we will need to create template file for our viewAction. In scripts/views/ directory create view.phtml file and place the following code in it.
Zend_Dojo_View_Helper_Dojo::setUseDeclarative();
$this->dojo()->setLocalPath('http://localhost/Zend/js/dojo/dojo/dojo.js')
->addStyleSheetModule(’dijit.themes.tundra’)
->addStylesheet('http://localhost/Zend/js/dojo/dojox/grid/_grid/tundraGrid.css');
echo $this->dojo();
<script type="text/javascript">
dojo.require("dojox.data.QueryReadStore");
dojo.require("dojox.grid.Grid");
dojo.require("dojo.parser");
</script>
<body class="tundra">
<div dojoType="dojox.data.QueryReadStore" jsId="activeStore", url="records"></div>
<div dojoType="dojox.grid.data.DojoData" jsId="model" rowsPerPage="20″ store="activeStore"></div>
<table id="activePastes" dojoType="dojox.grid.Grid" model="model" style="height:300px; width:700px;">
<thead>
<tr>
<th field="id">Id</th>
<th field="title">Title</th>
<th field="description">Description</th>
<th field="posted_date">Posted</th>
</tr>
</thead>
</table>
</body>
Keep in mind this is the main template file where we are creating our gird.
In the first statement I told zend to use declarative instead of programmatic behavior which is default. Next I set local path and add specific stylesheets. Then in the javascript code I used statement
Dojo.requre(’dojox.data.’) etc
To include specific dojo js modules.
In the body tag I specify a class “tundra”. This is important for the stylesheet I have added.
Next tow div’s and table are the main code for creating grid.
The first div fetch the records from the url specified. This url may be different if you fetch records form the other controller or source. Please specify valid url otherwise you will not get what you want.
The next div act as a bridge between our first div and the table. This div take data from the first div and give it to the table for display purposes.
Keep in mind that all the attributes of the div’s and table are compulsory. Removing any of the attribute may result in unvalid records or empty page. So define everything that I have defined. Once you become able to display your records, you can play with these attributes.
This seems to be a good tutorial.. But I have a question... Where is the code for the controller?? I can't see it... :S
ReplyDeleteHello and thank your for this tutorial,
ReplyDeletehowever it doesn't work anymore in newer Dojo versions.
For example i can't get this to work with Dojo1.2.0 because .Grid is depricated, it's .DataGrid now.
and the DojoData doesn't exist at all.
Anonymous said:
ReplyDelete> however it doesn't work anymore in newer Dojo versions.
That's right ... :(
> however it doesn't work anymore in newer Dojo versions
ReplyDeleteit's good to know that, does anyone has a solution with newer Dojo (v1.3.2) ?
thx
i can confirm that this works correctly in the latest version of zend framework (1.9)
ReplyDeleteOne note is that you should probably put an "exit();" if you are using a layout in zf, or the json that the records action returns will be incorrect (a whole html page).
ReplyDeleteModel should be Job not Jobs according to Best Practices from MVC Frameworks . Accrdingly File should be Job.php .
ReplyDeleteIf you are using $_db Object Once then why are you using getAdapter() to get $_db Next Time creating unnecessary overhead ?
very good is tutorial are dojo
ReplyDeleteHi,
ReplyDeleteI have been following your tutorials. I am learning both Zend Framework and Dojo at the same time. I am not getting any records from the database. I have exacly followed your tutorial with the same names of controller, form, and model. Please advise.
Thanks for good tutorials. Keep it up.
Welcome to the career facts site where you can learn all you wanted to know about career.
ReplyDeleteI tried this code but i am not getting the actual structure of grid , i am just getting the headings and data.
ReplyDeleteThis is not working, only displaying the data fetch from table but not moving into the template(i.e view.phtml).
ReplyDeleteplease help me......
I am just a new learner in Dojo. I know it is a nice example. However, I tried on Dojo 1.6 and found it is not working. Because of some changes in Dojo.
ReplyDeleteFinally, I reference the Official site and change my code in view.phtml as below and it works again(the table is for my DB). Perhaps it can help.
dojo.require("dojo.store.JsonRest");
dojo.require("dojo.store.Memory");
dojo.require("dojo.store.Cache");
dojo.require("dojox.grid.DataGrid");
dojo.require("dojo.data.ObjectStore");
dojo.require("dojo.data.ItemFileWriteStore");
dojo.ready(function(){
mystore = new dojo.data.ItemFileWriteStore({url: "records"});
grid = new dojox.grid.DataGrid({
store:mystore,
structure: [
//{name:"id", field:"id" },
{name:"Branch", field:"branch" },
{name:"Card#", field:"cardno"},
{name:"IOTime", field:"iotime", width: "200px"},
{name:"Staff#", field:"staffno", width: "200px"},
//{name:"Event", field:"eventid", width: "200px"},
{name:"IO", field:"io", width: "200px"},
{name:"Door", field:"doorname", width: "200px"}
]
}, "target-node-id"); // make sure you have a target HTML element with this id
grid.startup();
dojo.query("#save").onclick(function(){
dataStore.save();
});
});
Demo: Door Lock Data to DataGrid
Save
Sorry for I didn't tried the Edit. I just tested the display.
ReplyDeleteThis tutorial is way too old. Need some update. However, it is very helpful. Thank you.
ReplyDeleteUsually I do not column comments on blogs, but I would like to say that this blog absolutely affected me to do so! Thanks, for a absolutely nice read.
ReplyDeleteAdvertising agencies
Oracle fusion HCM Training from ERPTREE gives you the best results to learn your dream course and maintains sufficient knowledge on oracle. It provides training by self-paced videos which are very helpful for the users to watch at any time according to their schedule. It is globally accepted and having many users undergoing
ReplyDeletetraining every day.
Oracle fusion HCM Online Training
Oracle Fusion HCM Training
marketing companies in Islamabad
ReplyDeletemarketing company in Pakistan
real estate marketing company Islamabad
construction companies in Peshawar
DigisolHub
ReplyDeleteSearch Engine Optimization SEO is an organic method step by step that gives the website “search words” importance. SEO-friendly content which is written on the website can be optimised easily. If you want to rank your website on Google or Amazon, SEO experts plan and execute strategies in such a way that your content on websites gets more traffic. Usually, a website takes 4 to 6 months to get optimised. Here are the following tips which should be kept in mind in order to avoid SEO errors… read more
Thanks and I have a super proposal: Where To Learn Home Renovation home addition construction
ReplyDelete